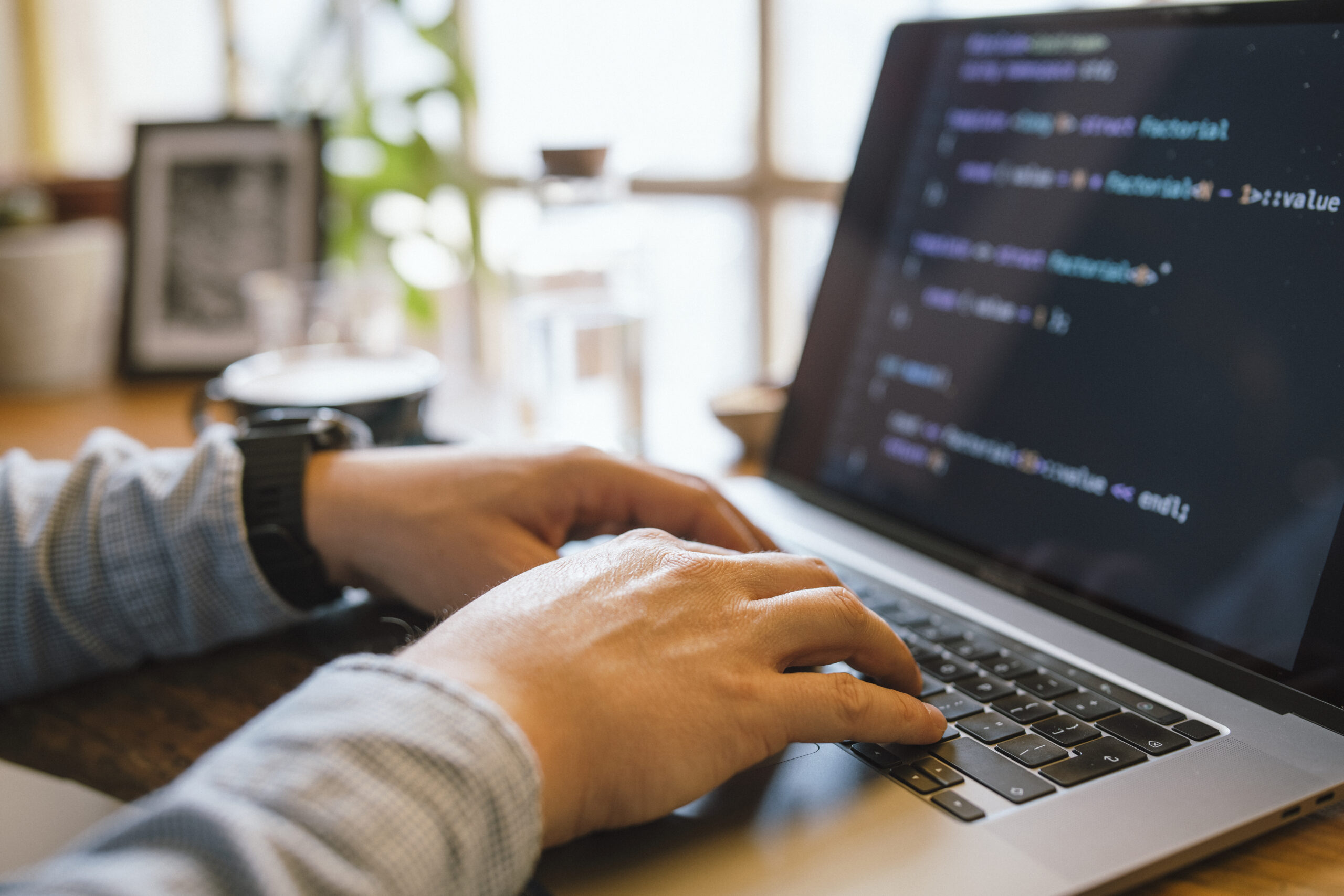
Debugging is Just about the most crucial — still often ignored — competencies in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging skills can save hours of frustration and dramatically improve your efficiency. Here i will discuss quite a few tactics that will help builders stage up their debugging match by me, Gustavo Woltmann.
Master Your Tools
One of the fastest approaches developers can elevate their debugging abilities is by mastering the applications they use everyday. When composing code is 1 part of enhancement, figuring out the way to interact with it effectively through execution is equally important. Fashionable growth environments come Geared up with highly effective debugging capabilities — but lots of builders only scratch the surface of what these instruments can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code about the fly. When used effectively, they let you notice exactly how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer applications, including Chrome DevTools, are indispensable for front-conclude builders. They enable you to inspect the DOM, observe network requests, watch genuine-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can switch frustrating UI concerns into workable tasks.
For backend or program-amount developers, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over managing procedures and memory management. Studying these applications could have a steeper learning curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Variation control methods like Git to grasp code heritage, obtain the precise moment bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments suggests likely further than default configurations and shortcuts — it’s about developing an intimate knowledge of your improvement atmosphere to ensure when difficulties occur, you’re not missing in the dead of night. The greater you already know your instruments, the greater time you may commit fixing the actual issue instead of fumbling via the process.
Reproduce the issue
One of the most critical — and infrequently missed — techniques in productive debugging is reproducing the challenge. Just before jumping into your code or earning guesses, builders want to create a constant environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a video game of possibility, frequently bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it results in being to isolate the exact conditions beneath which the bug occurs.
As soon as you’ve collected ample info, endeavor to recreate the issue in your neighborhood atmosphere. This might mean inputting the exact same information, simulating related user interactions, or mimicking program states. If The difficulty appears intermittently, take into account writing automated assessments that replicate the sting circumstances or point out transitions associated. These exams not simply help expose the challenge and also stop regressions Sooner or later.
From time to time, The difficulty might be setting-specific — it might come about only on selected operating methods, browsers, or beneath individual configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms is usually instrumental in replicating this kind of bugs.
Reproducing the trouble isn’t merely a action — it’s a frame of mind. It involves tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be already midway to correcting it. With a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and talk far more Plainly using your crew or end users. It turns an abstract complaint into a concrete challenge — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. In lieu of observing them as annoying interruptions, developers should learn to take care of error messages as direct communications from the process. They typically let you know precisely what transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by reading the information meticulously and in whole. A lot of developers, specially when below time pressure, look at the very first line and straight away start off creating assumptions. But further while in the error stack or logs may well lie the correct root induce. Don’t just copy and paste mistake messages into serps — go through and have an understanding of them to start with.
Split the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a particular file and line selection? What module or perform brought on it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically abide by predictable designs, and Discovering to recognize these can substantially speed up your debugging method.
Some glitches are vague or generic, and in People conditions, it’s essential to look at the context wherein the error occurred. Check out linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial challenges and provide hints about possible bugs.
Eventually, mistake messages are certainly not your enemies—they’re your guides. Learning to interpret them properly turns chaos into clarity, aiding you pinpoint troubles speedier, cut down debugging time, and become a much more effective and assured developer.
Use Logging Correctly
Logging is Among the most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an software behaves, helping you understand what’s happening underneath the hood without having to pause execution or move in the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging stages contain DEBUG, Information, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information and facts all through enhancement, Details for standard functions (like productive commence-ups), WARN for opportunity challenges that don’t split the application, Mistake for genuine troubles, and FATAL when the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and decelerate your process. Give attention to key situations, condition changes, enter/output values, and demanding conclusion factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler here to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically important in manufacturing environments where by stepping by means of code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-considered-out logging approach, it is possible to lessen the time it takes to spot difficulties, gain deeper visibility into your apps, and Increase the overall maintainability and reliability of the code.
Assume Like a Detective
Debugging is not only a complex endeavor—it is a type of investigation. To efficiently establish and take care of bugs, developers should technique the method similar to a detective solving a mystery. This attitude can help stop working elaborate issues into manageable components and stick to clues logically to uncover the basis lead to.
Start out by accumulating proof. Think about the indications of the problem: mistake messages, incorrect output, or performance problems. Much like a detective surveys a crime scene, collect as much relevant information as you are able to with out jumping to conclusions. Use logs, test instances, and user reports to piece alongside one another a transparent photograph of what’s going on.
Upcoming, sort hypotheses. Question by yourself: What may be leading to this behavior? Have any changes recently been built to your codebase? Has this challenge transpired prior to under identical situation? The purpose is always to narrow down alternatives and establish likely culprits.
Then, check your theories systematically. Try to recreate the condition in the controlled ecosystem. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, ask your code thoughts and Allow the results guide you nearer to the truth.
Pay back near focus to small facts. Bugs usually disguise while in the least envisioned areas—similar to a missing semicolon, an off-by-one mistake, or a race affliction. Be comprehensive and affected individual, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes might disguise the real dilemma, just for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging approach can help you save time for long term difficulties and help Other individuals fully grasp your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated programs.
Generate Tests
Creating exams is among the best tips on how to improve your debugging expertise and Total progress performance. Checks not only assist catch bugs early but additionally serve as a safety Internet that provides you self confidence when building variations towards your codebase. A well-tested software is much easier to debug mainly because it allows you to pinpoint precisely exactly where and when an issue occurs.
Start with device checks, which deal with person functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to glimpse, noticeably cutting down enough time put in debugging. Unit checks are Primarily handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Following, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance be sure that a variety of elements of your software operate with each other smoothly. They’re specially beneficial for catching bugs that occur in elaborate techniques with numerous parts or providers interacting. If something breaks, your assessments can tell you which part of the pipeline unsuccessful and under what ailments.
Creating assessments also forces you to Assume critically about your code. To check a function thoroughly, you will need to understand its inputs, anticipated outputs, and edge cases. This amount of understanding In a natural way potential customers to higher code composition and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a strong starting point. Once the examination fails continuously, you'll be able to center on fixing the bug and observe your take a look at go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the problem—looking at your display for hrs, hoping Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you are also close to the code for also extended, cognitive fatigue sets in. You may begin overlooking apparent errors or misreading code that you wrote just hours before. With this condition, your brain turns into much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a distinct activity for 10–quarter-hour can refresh your aim. A lot of developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, In particular for the duration of for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not only unproductive but will also draining. Stepping away allows you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weakness—it’s a wise strategy. It provides your Mind House to breathe, improves your viewpoint, and helps you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of resolving it.
Learn From Every single Bug
Each individual bug you encounter is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something beneficial should you make the effort to replicate and review what went wrong.
Begin by asking oneself a number of critical issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The answers often expose blind places as part of your workflow or being familiar with and assist you Establish much better coding behaviors transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. With time, you’ll start to see styles—recurring difficulties or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is often Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as essential portions of your improvement journey. In fact, a number of the most effective developers are usually not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, each bug you correct provides a fresh layer on your skill set. So upcoming time you squash a bug, take a second to mirror—you’ll occur away a smarter, far more able developer due to it.
Conclusion
Increasing your debugging abilities normally takes time, observe, and patience — nevertheless the payoff is large. It makes you a more productive, self-confident, and able developer. The next time you're knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.